The Developers
I've been reading this great article from Reactjs's new documentation: https://react.dev/learn/you-might-not-need-an-effect which Dan Abramov tweeted about a while back.

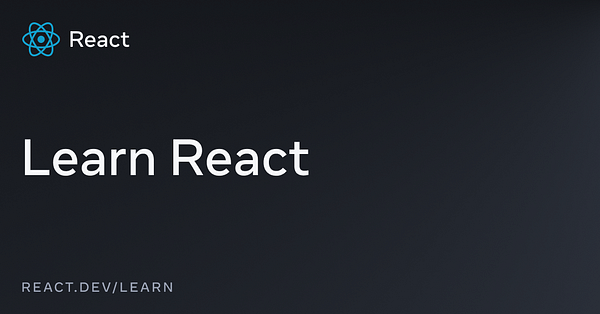
He mentions that he found many unnecessary uses of React's useEffect hook in Facebook’s codebase, like a big percentage of total useEffect uses was "useless", pun intended. In my opinion, this actually points to a flaw in the framework itself rather than the developers’ use of it. If you needed that much documentation and posts about how to useEffect after however many years from it being released, then there’s something wrong.
There was also a big discussion on twitter recently about Signals and how React’s components need a lot of useMemo and useCallback because if you calculate derived state on every render and your calculation is slow then you need to cache everything. Not saying that Signals are a good solution.
The Real World
I’ve been trying to write a complex UI for a project that I’m developing to help my wife in her research. Apparently, writing complex UI in react sometimes becomes a nightmare. I found myself writing a lot of the bad examples in the linked article. After reading the article I went back and modified my code and it became much shorter and better. But, that wasn’t as clear before to me. And still, I’m not very satisfied with how React is mostly doing very inefficient things to the web. Immutability in JS is very inefficient (compared to FP languages for example e.g. Haskell) and that turns many websites into very slow websites. The problem is very obvious on older hardware.
const [todos, setTodos] = useState(["Todo 1", "Todo 2"]);
...
<button onClick={() => setTodos(todos.filter(t => t === todo))}>Remove Todo</button>
Just look at this example, to remove a todo you don’t only have to get through the whole list of todos, but if your items are just a bunch of strings, the equality check has to go through the length of the strings it’s comparing. This is very bad and when you have tens or hundreds of components in a page, the problem isn’t so easy to spot. Even if you say you should profile, so many people don’t profile because the website always feels fast on their modern machine. But I’m 100% sure that lots of people around the world carry very old hardware that would make a react website much slower than how a developer sees it.
Adding to a list state involves copying the whole list, adding to object needs copying the object … etc:
setTodos([...todos, newTodo])
setState({...state, newProp: _})
setSomeMap(new Map(someMap.set('someKey', 'a new value')));
// ...
// you get it
And of course for react this is all fine because having a view as a function of the state is good enough even if it doesn’t suit the performance characteristics of the hosting language.
The Code
Back to my problem, I was mainly trying to do a custom WYSIWYG editor that you can tag text in with some custom UI, I chose TipTap editor (a famous library for ProseMirror in react) because it’s famous and it had a nice and simple documentation that I thought would be helpful. The editor is amazing but because react doesn’t play nicely with things that need to live outside of its control e.g. ProseMirror , it felt like I was hitting bug after bug in how TipTap was implementing the react part of the editor. It was very frustrating and I still have issues that I couldn’t find a workaround for. Look at all the React hooks that most of the developers don’t know about but has to be used in TipTap to get React to work nicely with ProseMirror. My options are now limited to switching to some other editor library or get to use ProseMirror directly without TipTap or …
Rewrite Everything in X
Svelte, is something that I got to try recently after watching Rich Harris teaching it on YouTube (of course I knew about Svelte before, but the recently release SvelteKit looked even better and the YouTube video was the final push for me). So given that I enjoy trying new tech more than working on actual projects™, I thought why not rewrite everything in Svelte™ (maybe we should start that movement in the frontend world à la rewrite everything in Rust). Svelte is a breath of fresh air coming from React. It’s all about web standards, the reactivity system is beautiful, mutation and respecting the performance of the hosting language is there and SvelteKit’s actions are amazing (like Remix’s) and the editor was a breeze to implement. Look even TipTap doesn’t have a specific Svelte implementation, they just tell you install TipTap core module and write Svelte code. The best thing is it would work perfectly fine with ProseMirror if I wanted to because Svelte doesn’t have a problem with things that live outside of Svelte’s control.
I don’t wish for React to die but React is bad for the web, for developers, and for people who browse the internet. So I actually think I want React to die and we all use Svelte, lol.
I enjoy trying new tech more than working on actual projects
— Mohamed Elsharnouby